Introduction
russia’s massive attacks on Ukraine’s energy infrastructure have led to large-scale power outages (blackouts). This has created everyday problems for ordinary civilians, including: teaching children in the evening without electricity, moving elderly people around in high-rise buildings, cooking food using electric stoves, storing food in the refrigerator, heating water with a boiler, air conditioning rooms, and other problems associated with the lack of power supply.
Unfortunately, I also suffered from this fate, which motivated me to create a system that would notify me of the time and duration of the power outage in order to be able to predict the duration of the next outage. This would allow me to determine which generator or charging station to buy in the future to cover the minimum needs in the absence of centralized power supply.
Infrastructure
To implement my idea, I had previously created an infrastructure that fully met my needs, namely:
- Any device that is connected to the power grid and local network via a wired connection or via wi-fi.
- Router, switch and wi-fi access point that are connected to both the network and an additional power source
- Raspberry Pi that is also connected to an alternative power source
In my case, the role of the home device was a wi-fi thermostat for underfloor heating. The role of the network devices was played by devices from Ubiquiti, and the role of data processing was played by my Raspberry Pi.
I depicted the principle of connection and interaction graphically
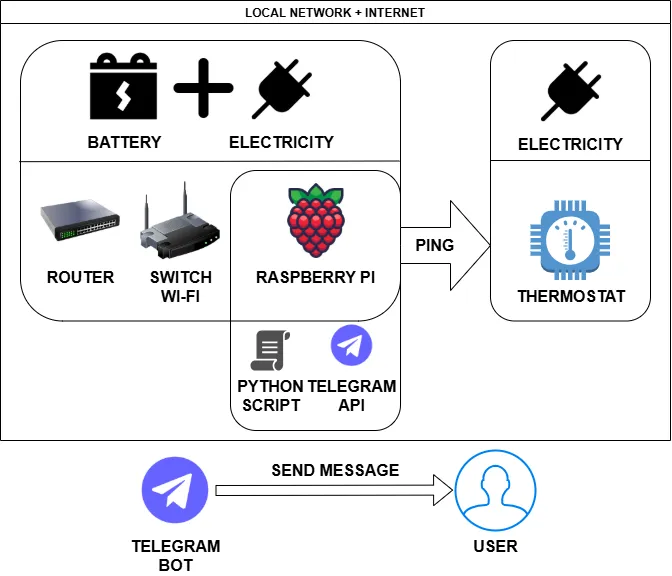
That is, a script written in Python constantly sends ping requests 24/7. If the electricity is out, then of course the thermostat will not be able to respond, but the network equipment and Raspberry Pi will continue to work from an alternative energy source, and the user will learn about the lack of electricity via a Telegram push notification. When the electricity is restored, the thermostat will appear on the local network, and if the ping is successfully answered, the user will be informed that electricity has appeared, and the time of the power outage will also be calculated.
- To implement this algorithm, you need:
- Creating a Telegram bot
- Writing a Python script
- Automating startup via a service
I will describe these points in detail.
Creating a Telegram bot
There is a special bot in Telegram that is created specifically for creating other bots. This bot is called BotFather. To create your own bot, you need to perform the following steps:
- You need to find a bot called BotFather in Telegram
- To get started, you need to write /start
- To create your bot, you need to enter the command /newbot
- Enter the name and title of your bot
After creating the bot, you can set privacy and get an API token through the main menu. You also need to get a Chat_ID for private use of the bot, because it needs to know who to send messages to. For this, there is another bot @userinfobot, you need to write something to it, for example /start and it will write a Chat_ID in response, which will be used in the future.
The most important thing we got:
- API token
- Chat_ID
Let’s move on to creating a script in Python.
Writing a Python script
To run the script, you need to install additional packages and libraries, create and activate a virtual environment.
Installing additional packages
sudo apt install python3-venv
Creating a virtual environment in the desired directory, in my case /home/rpi/TG_BOT
cd /home/rpi/TG_BOT
python3 -m venv myenv
Activating a virtual environment
source myenv/bin/activate
Once the virtual environment is activated, you need to install libraries to work with the Telegram API.
pip install python-telegram-bot requests
СThe script will have the following functions:
- format_time – to determine the time
- send_message – to send a message
- ping_address – to ping the thermostat
- monitor_ip – to determine the status and generate a message
Code provided in the spoiler
electricity.py
import os
import time
import asyncio
from telegram import Bot
CHAT_ID = "<Your Chat ID>"
BOT_TOKEN = "<Your API tioken>"
bot = Bot(token=BOT_TOKEN)
def format_time(seconds):
hours, remainder = divmod(seconds, 3600)
minutes, seconds = divmod(remainder, 60)
return f"{hours} hours {minutes} minutes {seconds} seconds"
async def send_message(message):
await bot.send_message(chat_id=CHAT_ID, text=message)
def ping_address(ip, count=3):
response = os.system(f"ping -c {count} -W 2 {ip} | ts")
return response == 0
async def monitor_ip(ip):
power_off_time = None
power_on = True
while True:
if ping_address(ip):
if not power_on:
power_on = True
time_power_on = time.time()
outage_duration = int(time_power_on - power_off_time)
formatted_duration = format_time(outage_duration)
outage_message = f"The light came on. The blackout continued {formatted_duration}."
await send_message(outage_message)
else:
if power_on:
power_on = False
power_off_time = time.time()
await send_message("The light disappeared.")
await asyncio.sleep(30)
if __name__ == "__main__":
ip_address = "<Thermostat local IP>"
asyncio.run(monitor_ip(ip_address))
Usually, the command execution log is written to the system log, and each entry there has a date and time stamp, but I decided to separate the logging into a separate file using the service. If logging does not occur in the system log, then by default the event time is not recorded. In order for the ping time to be recorded, I additionally process the ping command with the ts command, thus the output of the entry:
ping -c 3 8.8.8.8
PING 8.8.8.8 (8.8.8.8) 56(84) bytes of data.
64 bytes from 8.8.8.8: icmp_seq=1 ttl=118 time=16.1 ms
turns into:
ping -c 3 8.8.8.8 | ts
May 12 19:18:23 PING 8.8.8.8 (8.8.8.8) 56(84) bytes of data.
May 12 19:18:23 64 bytes from 8.8.8.8: icmp_seq=1 ttl=118 time=15.7 ms
To do this, you need to additionally install the moreutils utility.
Automating startup via service
To ensure that the script runs even after the Raspberry Pi reboots, I created a service that will start automatically. Usually, all system services are located in the /etc/systemd/system/ directory, so I will place the service for the script there as well. As I mentioned earlier, logging will be done in a separate file for convenience, at the address /var/log/sun.log, so this address will be specified in the service parameters.
I came up with the following name for my service – FolletSun.service. The service parameters will be as follows:
[Unit]
Description=Script which check electricity and pass status to Telegram Bot.
[Service]
ExecStart=/home/rpi/TG_BOT/bin/python3 /home/rpi/TG_BOT/Sun.py
StandardOutput=file:/var/log/sun.log
StandardError=file:/var/log/sun.log
WorkingDirectory=/home/rpi/TG_BOT
Restart=always
User=rpi
Group=rpi
[Install]
WantedBy=multi-user.target
In order to initiate the service without rebooting the system, you must execute the following commands:
sudo systemctl daemon-reexec
sudo systemctl daemon-reload
Also, to activate the service, start it, and check its status, run the following commands:
sudo systemctl enable FolletSun.service
sudo systemctl start FolletSun.service
sudo systemctl status FolletSun.service
The result of executing the service status command will be as follows:
● FolletSun.service - Script which check electricity and pass status to Telegram Bot.
Loaded: loaded (/etc/systemd/system/FolletSun.service; enabled; preset: enabled)
Active: active (running) since Sun 2025-05-11 22:31:20 EEST; 21h ago
Main PID: 1458494 (python3)
Tasks: 1 (limit: 8735)
CPU: 3min 40.583s
CGroup: /system.slice/FolletSun.service
└─1458494 /home/rpi/TG_BOT/bin/python3 /home/rpi/TG_BOT/Sun.py
This indicates that no errors or conflicts were found in the script.
Checking the bot’s operation
To check the bot’s operation, you need to find the created bot in the Telegram application and subscribe to it. From this moment on, the bot should notify about the change in the power supply status. Here is a screenshot from my smartphone:
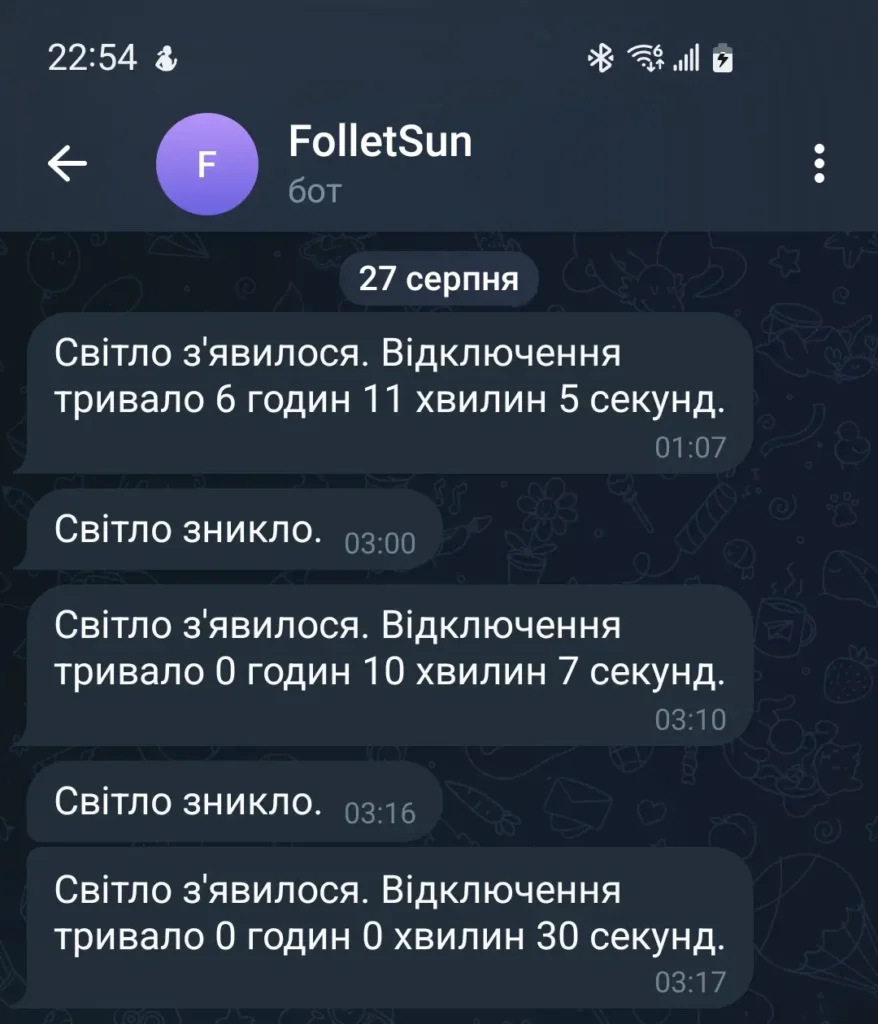
The ping occurs every 30 seconds, and you probably noticed a message where the power went out for only 30 seconds – this is a false alarm because the thermostat did not respond to the ping request, or the Raspberry Pi did not receive a response from the thermostat. This may be the result of an unstable connection. In order to avoid this or minimize it, it is better to use at least 3 ping requests.
Conclusions
In response to the current threats related to energy security in wartime, an effective and inexpensive power supply monitoring system was developed. It is based on popular open source technologies – the Raspberry Pi microcomputer, Python scripts and Telegram bots.
The system provides continuous monitoring of the availability of electricity in the house, records the time of its disappearance and return, and also automatically sends notifications to the user. This approach allows you to quickly respond to the situation, plan the use of backup power sources and make informed decisions about purchasing a generator or charging station.
The project proves that with existing infrastructure and basic programming knowledge, you can independently create a useful tool for increasing comfort and safety in critical conditions. The proposed solution is scalable, easy to maintain, and can be adapted to any other home device or object that is connected to the network.